This content originally appeared on DEV Community and was authored by Matan Shaviro
In modern JavaScript development, handling asynchronous operations efficiently is essential, especially when multiple tasks need to run simultaneously. That’s where Promise.all comes in—a powerful method that allows you to execute several promises at once, waiting for all of them to resolve (or for one to reject) before proceeding. In this post, we’ll dive into how Promise.all works, why it’s such a valuable tool for managing concurrent asynchronous operations, and how you can implement it yourself to deepen your understanding of JavaScript promises.
Let’s get started!
Example usage
const p1 = Promise.resolve(1)
const p2 = Promise.resolve(2)
const p3 = Promise.resolve(3)
customPromiseAll([p1, p2, p3])
.then((res) => console.log(res))
.catch(console.error)
When we start writing the function, in order for the Promise to work at all, we’ll first return a new instance of Promise like this:
function customPromiseAll(promises) {
return new Promise((resolve, reject) => {
})
}
Now, we’ll create a variable to hold the result of the function. It will be an array, and we’ll call it result. Additionally, we’ll create a variable named completed to keep track of the number of promises that have been completed.
function customPromiseAll(promises) {
return new Promise((resolve, reject) => {
const results = []
let completed = 0
})
}
We’ll check an edge case: if the array of promises the function receives is empty, we’ll return an empty array as a resolved value and finish.
function customPromiseAll(promises) {
return new Promise((resolve, reject) => {
const results = []
let completed = 0
if (promises.length === 0) {
resolve([])
}
})
}
If not, we’ll iterate over the array of promises the function receives using forEach and handle each resolve like this:
function customPromiseAll(promises) {
return new Promise((resolve, reject) => {
const results = []
let completed = 0
if (promises.length === 0) {
resolve([])
}
promises.forEach((promise, index) => {
Promise.resolve(promise)
.then((value) => {
})
})
})
}
We’ll store each value in the results array at its designated index and increment completed by 1.
function customPromiseAll(promises) {
return new Promise((resolve, reject) => {
const results = []
let completed = 0
if (promises.length === 0) {
resolve([])
}
promises.forEach((promise, index) => {
Promise.resolve(promise)
.then((value) => {
results[index] = value
completed++
})
})
})
}
Now, we’ll check if completed is equal to the length of the promises array. If it is, we’ve gone through all the items in the array, which means we’re done, so we’ll resolve the results array.
function customPromiseAll(promises) {
return new Promise((resolve, reject) => {
const results = []
let completed = 0
if (promises.length === 0) {
resolve([])
}
promises.forEach((promise, index) => {
Promise.resolve(promise)
.then((value) => {
results[index] = value
completed++
if (completed === promises.length) {
resolve(results)
}
})
})
})
}
Finally, we’ll add .catch in case any of the promises return an error.
function customPromiseAll(promises) {
return new Promise((resolve, reject) => {
const results = []
let completed = 0
if (promises.length === 0) {
resolve([])
}
promises.forEach((promise, index) => {
Promise.resolve(promise)
.then((value) => {
results[index] = value
completed++
if (completed === promises.length) {
resolve(results)
}
})
.catch(reject) // Reject immediately if any promise fails
})
})
}
This content originally appeared on DEV Community and was authored by Matan Shaviro
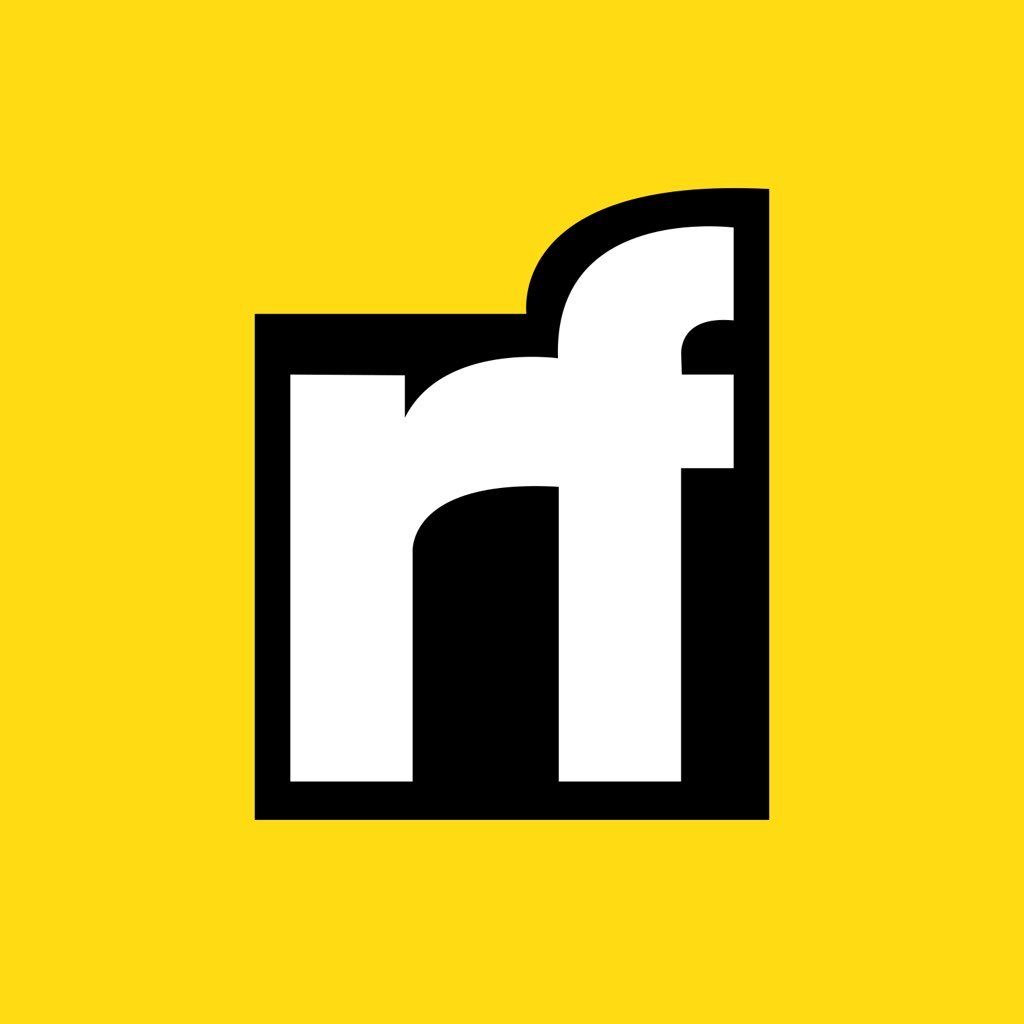
Matan Shaviro | Sciencx (2024-11-12T08:39:11+00:00) Your own Promise.all. Retrieved from https://www.scien.cx/2024/11/12/your-own-promise-all/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.