This content originally appeared on DEV Community and was authored by emojiiii
Background removal is a common task in image processing that has traditionally required complex desktop software or cloud-based services. However, with recent advances in web technologies and AI models, it's now possible to build a powerful background remover that runs entirely in the browser. In this tutorial, we'll explore how to create such a tool using React, Transformers.js, and state-of-the-art AI models.
Key Features
- 🚀 Client-side processing - no server uploads needed
- 🎯 Support for multiple AI models (RMBG-1.4 and ModNet)
- 📦 Batch processing capabilities
- 🎨 Built-in image editor for post-processing
- đź”’ Privacy-focused - all processing happens locally
Technical Architecture
The application is built with several key components:
- Frontend UI: React with TypeScript for type safety
- AI Processing: Transformers.js for running AI models
- Worker Thread: Web Workers for non-blocking processing
- State Management: React hooks for local state management
Implementation Details
Setting Up the Models
We use two different models for background removal:
type ModelType = "briaai/RMBG-1.4" | "Xenova/modnet";
RMBG-1.4 is our recommended model for better quality, while ModNet serves as an alternative option. Both models are loaded and run entirely in the browser using Transformers.js.
Core Components
The main component structure consists of three key areas:
- Upload Area: Handles file input and model selection
- Edit Area: Displays the processed image with editing capabilities
- Image List: Shows all uploaded images and their processing status
Worker Thread Implementation
To keep the UI responsive during image processing, we use a Web Worker:
const useTask = (onImageProcessed?: (id: string) => void) => {
const [files, setFiles] = useState<FileWithMoreInfo[]>([]);
const { worker, isModelLoading } = useWorker(
(event: WorkerResponseMessageEvent) => {
const { type, data, id, status } = event.data;
switch (type) {
case WorkerResponseTaskType.REMOVE_BACKGROUND_COMPLETE:
// Update UI with processed image
break;
}
}
);
// ... task management logic
};
Processing Pipeline
- User uploads an image(s)
- Images are queued for processing
- Worker thread loads the selected AI model
- Background removal is performed
- Processed images are displayed with transparent backgrounds
Post-Processing Features
After background removal, users can:
- Rotate the image
- Add text or stickers
- Apply filters
- Download individual images or batch download as ZIP
Performance Considerations
- Models are cached after first load
- Processing happens in chunks to prevent UI freezing
- Images are processed sequentially in batch uploads
- Preview thumbnails are generated efficiently
Future Improvements
- Support for more AI models
- Advanced editing features
- Background replacement options
- Batch processing optimization
- Export in different formats
Conclusion
Building a browser-based background remover demonstrates how far web technologies have come. By leveraging modern frameworks and AI models, we can create powerful image processing tools that run entirely on the client side, ensuring both performance and privacy.
The complete source code showcases how to structure such an application, handle complex image processing tasks, and provide a smooth user experience. Feel free to explore and adapt this implementation for your own projects!
Resources
This content originally appeared on DEV Community and was authored by emojiiii
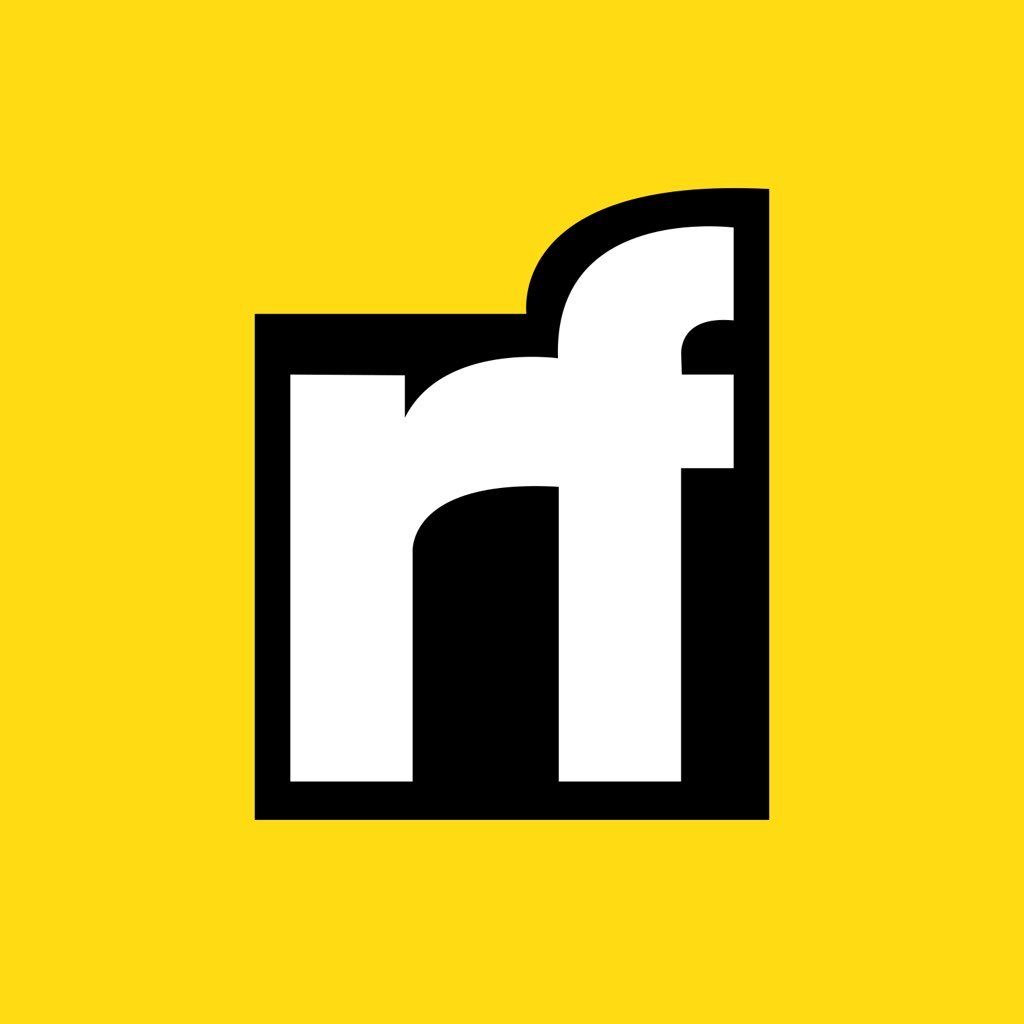
emojiiii | Sciencx (2025-01-12T07:01:37+00:00) Building an AI-Powered Background Remover with React and Transformers.js. Retrieved from https://www.scien.cx/2025/01/12/building-an-ai-powered-background-remover-with-react-and-transformers-js/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.