This content originally appeared on DEV Community and was authored by Lucky Jain
Hey there, developers! Lucky Jain here, and today we’re diving into the world of state management in React. If you’ve ever felt lost in the maze of props drilling or overwhelmed by the complexities of state management tools, I’ve got your back. Let’s break it down step by step in the simplest way possible.
Why Do We Need State Management?
React is great for building interactive UIs, but when your app starts growing, so does the complexity of managing state. Passing props down multiple levels (props drilling) can quickly turn into a nightmare. Enter state management solutions to save the day!
There are many options out there, but today, we’ll focus on two popular choices: Context API and Redux Toolkit.
1. Context API: The Built-In Hero
The Context API is React’s built-in solution for managing state. It’s perfect for apps where you don’t need a lot of bells and whistles.
When to Use Context API
- Sharing data like themes, user authentication, or language preferences.
- Small to medium-sized apps.
How It Works
Think of Context API as a way to create a global variable that any component in your app can access.
Code Example
Here’s a quick example of using Context API to manage a theme:
import React, { createContext, useContext, useState } from "react";
// 1. Create Context
const ThemeContext = createContext();
// 2. Create a Provider Component
const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState("light");
const toggleTheme = () => {
setTheme((prevTheme) => (prevTheme === "light" ? "dark" : "light"));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
};
const App = () => {
return (
<ThemeProvider>
<Home />
</ThemeProvider>
);
};
const Home = () => {
const { theme, toggleTheme } = useContext(ThemeContext);
return (
<div style={{ background: theme === "light" ? "#fff" : "#333", color: theme === "light" ? "#000" : "#fff" }}>
<h1>{theme.toUpperCase()} MODE</h1>
<button onClick={toggleTheme}>Toggle Theme</button>
</div>
);
};
export default App;
Pros
- Simple and easy to use.
- No extra libraries needed.
Cons
- Can lead to performance issues with deeply nested components.
- Not ideal for complex state logic.
2. Redux Toolkit: The Powerhouse
For larger, more complex apps, Redux Toolkit is a game-changer. It’s a modern way to use Redux without the boilerplate.
When to Use Redux Toolkit
- Apps with complex state logic.
- When you need features like middleware for async actions.
How It Works
Redux Toolkit simplifies the Redux setup by bundling the essential tools into a single package.
Code Example
Let’s build a simple counter app using Redux Toolkit:
1. Install Redux Toolkit
npm install @reduxjs/toolkit react-redux
2. Create a Slice
// src/features/counter/counterSlice.js
import { createSlice } from "@reduxjs/toolkit";
const counterSlice = createSlice({
name: "counter",
initialState: { value: 0 },
reducers: {
increment: (state) => { state.value += 1; },
decrement: (state) => { state.value -= 1; },
reset: (state) => { state.value = 0; },
},
});
export const { increment, decrement, reset } = counterSlice.actions;
export default counterSlice.reducer;
3. Configure the Store
// src/app/store.js
import { configureStore } from "@reduxjs/toolkit";
import counterReducer from "../features/counter/counterSlice";
const store = configureStore({
reducer: {
counter: counterReducer,
},
});
export default store;
4. Use Redux in Components
// src/App.js
import React from "react";
import { useSelector, useDispatch } from "react-redux";
import { increment, decrement, reset } from "./features/counter/counterSlice";
const App = () => {
const count = useSelector((state) => state.counter.value);
const dispatch = useDispatch();
return (
<div style={{ textAlign: "center" }}>
<h1>Counter: {count}</h1>
<button onClick={() => dispatch(increment())}>Increment</button>
<button onClick={() => dispatch(decrement())}>Decrement</button>
<button onClick={() => dispatch(reset())}>Reset</button>
</div>
);
};
export default App;
Pros
- Handles complex state logic efficiently.
- Great dev tools and debugging capabilities.
- Built-in support for async actions (via
createAsyncThunk
).
Cons
- Slightly more setup than Context API.
Context API vs Redux Toolkit: Which One Should You Choose?
Here’s a quick comparison to help you decide:
Feature | Context API | Redux Toolkit |
---|---|---|
Setup Complexity | Low | Moderate |
Performance | Can degrade with deep updates | Optimized for large apps |
Best Use Case | Small apps or shared data | Complex state logic apps |
Final Thoughts
State management doesn’t have to be scary. For small apps, stick with Context API. For larger apps, go for Redux Toolkit. And remember, there’s no one-size-fits-all solution. Choose what works best for your app.
Got questions or need help? Drop a comment below. Until next time, happy coding!
This content originally appeared on DEV Community and was authored by Lucky Jain
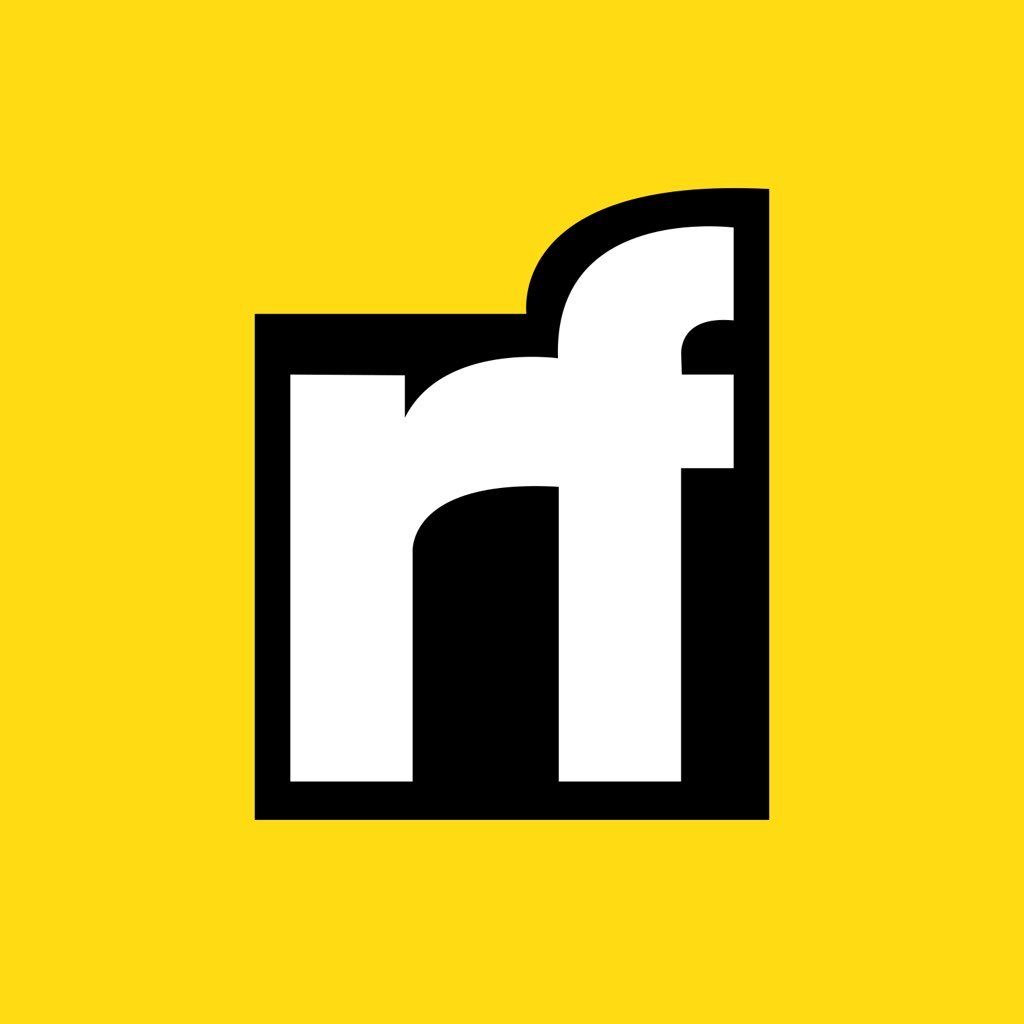
Lucky Jain | Sciencx (2025-01-25T22:19:01+00:00) All you need to know about state management in React!. Retrieved from https://www.scien.cx/2025/01/25/all-you-need-to-know-about-state-management-in-react/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.