This content originally appeared on DEV Community and was authored by Muhammad Usman
Mutator Methods (Modify the original array)
-
push(element1, ..., elementN)
Adds elements to the end. Returns: New length.
arr.push(5); // [1,2,3] → [1,2,3,5]
-
pop()
Removes the last element. Returns: Removed element.
arr.pop(); // [1,2,3] → [1,2]
-
shift()
Removes the first element. Returns: Removed element.
arr.shift(); // [1,2,3] → [2,3]
-
unshift(element1, ..., elementN)
Adds elements to the start. Returns: New length.
arr.unshift(0); // [1,2,3] → [0,1,2,3]
-
splice(start, deleteCount, ...items)
Adds/removes elements at start index. Returns: Array of removed elements.
arr.splice(1, 1, 'a'); // [1,2,3] → [1,'a',3]
-
sort([compareFunction])
Sorts elements (lexicographically by default). Returns: Sorted array (mutates original).
arr.sort((a, b) => a - b); // [3,1,2] → [1,2,3]
-
reverse()
Reverses the array. Returns: Reversed array (mutates original).
arr.reverse(); // [1,2,3] → [3,2,1]
-
fill(value, start?, end?)
Fills array withvalue
betweenstart
andend
.
arr.fill(0); // [1,2,3] → [0,0,0]
-
copyWithin(target, start, end?)
Copies elements within the array.
[1,2,3,4].copyWithin(0, 2); // → [3,4,3,4]
Accessor Methods (Return new data without modifying the array)
- concat(...arrays) Merges arrays. Returns: New merged array.
arr.concat([4,5]); // [1,2,3] → [1,2,3,4,5]
-
slice(start?, end?)
Returns subarray betweenstart
andend
.
arr.slice(1, 3); // [1,2,3,4] → [2,3]
-
join(separator?)
Joins elements into a string (default:,
).
arr.join('-'); // [1,2,3] → "1-2-3"
-
includes(element)
Checks if array containselement
. Returns:true
/false
.
arr.includes(2); // [1,2,3] → true
-
indexOf(element)
Returns first index ofelement
or-1
.
arr.indexOf(2); // [1,2,3] → 1
-
lastIndexOf(element)
Returns last index ofelement
or-1
.
[1,2,2,3].lastIndexOf(2); // → 2
-
toString()
Same asjoin()
.
[1,2,3].toString(); // "1,2,3"
-
toSorted() (ES2023)
Non-mutating version ofsort()
.
const sorted = arr.toSorted();
-
toReversed() (ES2023)
Non-mutating version ofreverse()
.
const reversed = arr.toReversed();
Iteration Methods
-
forEach(callback)
Executescallback
for each element. Returns:undefined
.
arr.forEach(x => console.log(x));
-
map(callback)
Returns new array with transformed elements.
const doubled = arr.map(x => x * 2);
- filter(callback)
Returns elements that pass
callback
test.
const evens = arr.filter(x => x % 2 === 0);
-
reduce(callback, initialValue?)
Reduces array to a single value.
const sum = arr.reduce((acc, x) => acc + x, 0);
-
find(callback)
Returns first element passingcallback
test.
const firstEven = arr.find(x => x % 2 === 0);
-
some(callback)
Checks if at least oneelement
passes test. Returns:true
/false
.
const hasEven = arr.some(x => x % 2 === 0);
-
every(callback)
Checks if all elements pass test.
const allEven = arr.every(x => x % 2 === 0);
-
flat(depth = 1)
Flattens nested arrays.
[1, [2]].flat(); // → [1,2]
- flatMap(callback) Maps then flattens the result.
arr.flatMap(x => [x, x*2]); // [1,2] → [1,2,2,4]
Static Methods
-
Array.isArray(value)
Checks if value is an array.
Array.isArray([1,2]); // → true
-
Array.from(arrayLike)
Creates an array from array-like/iterable.
Array.from('123'); // → ['1','2','3']
-
Array.of(...elements)
Creates an array from arguments.
Array.of(1,2,3); // → [1,2,3]
ES6+ Additions
-
at(index)
Returns element at index (supports negatives).
[1,2,3].at(-1); // → 3
-
findLast(callback) (ES2023)
Returns last element passing test.
[1,2,2,3].findLast(x => x === 2); // → 2
Key Notes:
-
Mutators: Modify the original array (e.g.,
push
,sort
). -
Accessors: Return new data without changing the original (e.g.,
slice
,concat
). -
Iterators: Process elements via callbacks (e.g.,
map
,filter
). -
Use toSorted
(),toReversed()
, etc., for non-mutating operations (ES2023+).
This content originally appeared on DEV Community and was authored by Muhammad Usman
Print
Share
Comment
Cite
Upload
Translate
Updates
There are no updates yet.
Click the Upload button above to add an update.
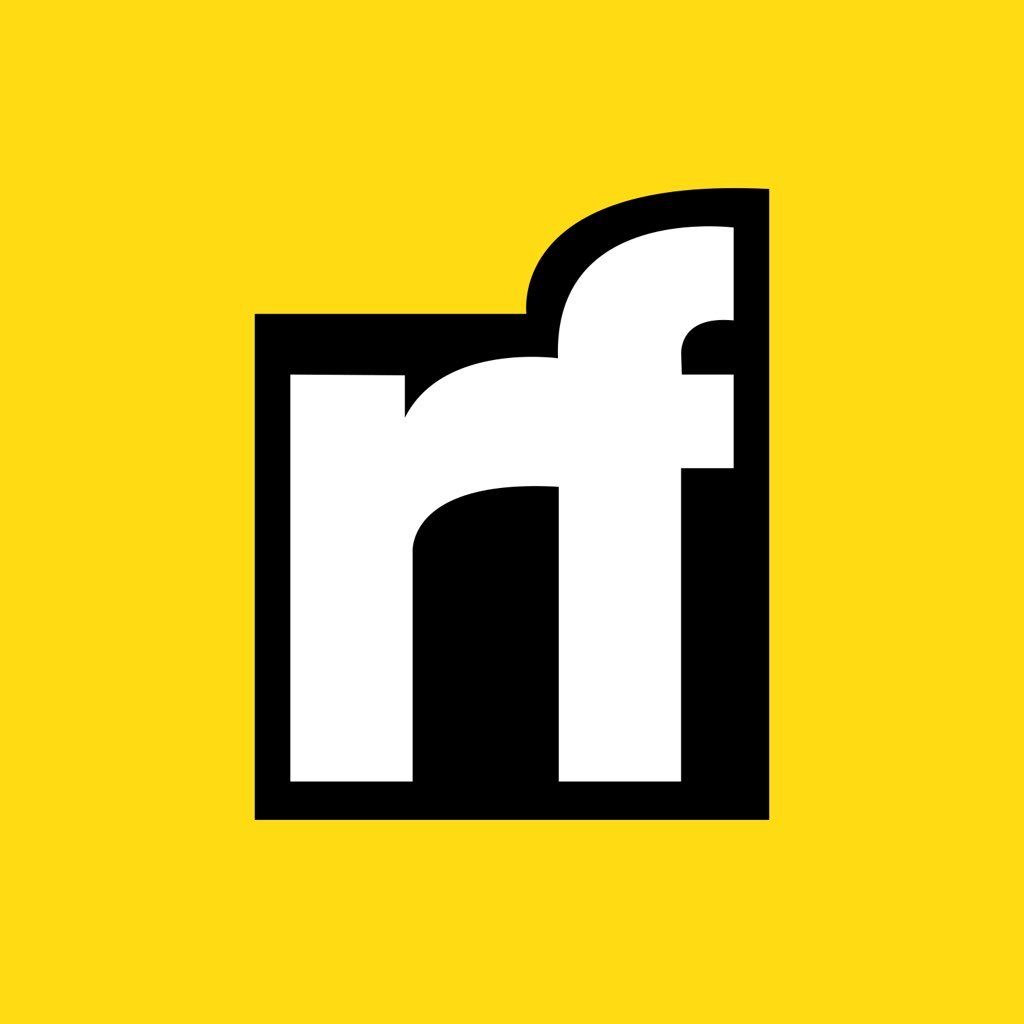
APA
MLA
Muhammad Usman | Sciencx (2025-02-06T05:38:53+00:00) JavaScript Array Methods Cheat Sheet: The Only Guide You Need. Retrieved from https://www.scien.cx/2025/02/06/javascript-array-methods-cheat-sheet-the-only-guide-you-need/
" » JavaScript Array Methods Cheat Sheet: The Only Guide You Need." Muhammad Usman | Sciencx - Thursday February 6, 2025, https://www.scien.cx/2025/02/06/javascript-array-methods-cheat-sheet-the-only-guide-you-need/
HARVARDMuhammad Usman | Sciencx Thursday February 6, 2025 » JavaScript Array Methods Cheat Sheet: The Only Guide You Need., viewed ,<https://www.scien.cx/2025/02/06/javascript-array-methods-cheat-sheet-the-only-guide-you-need/>
VANCOUVERMuhammad Usman | Sciencx - » JavaScript Array Methods Cheat Sheet: The Only Guide You Need. [Internet]. [Accessed ]. Available from: https://www.scien.cx/2025/02/06/javascript-array-methods-cheat-sheet-the-only-guide-you-need/
CHICAGO" » JavaScript Array Methods Cheat Sheet: The Only Guide You Need." Muhammad Usman | Sciencx - Accessed . https://www.scien.cx/2025/02/06/javascript-array-methods-cheat-sheet-the-only-guide-you-need/
IEEE" » JavaScript Array Methods Cheat Sheet: The Only Guide You Need." Muhammad Usman | Sciencx [Online]. Available: https://www.scien.cx/2025/02/06/javascript-array-methods-cheat-sheet-the-only-guide-you-need/. [Accessed: ]
rf:citation » JavaScript Array Methods Cheat Sheet: The Only Guide You Need | Muhammad Usman | Sciencx | https://www.scien.cx/2025/02/06/javascript-array-methods-cheat-sheet-the-only-guide-you-need/ |
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.