This content originally appeared on DEV Community and was authored by Pentest Testing Corp
Introduction
Business logic vulnerabilities pose significant threats to web applications, allowing attackers to exploit legitimate functionalities for malicious purposes.
In Laravel applications, these vulnerabilities can lead to unauthorized actions, data breaches, and financial losses.
This article explores common business logic vulnerabilities in Laravel and provides practical coding examples to help developers identify and prevent them.
What Are Business Logic Vulnerabilities?
Business logic vulnerabilities arise from flaws in an application's design and workflow, enabling attackers to manipulate processes to achieve unintended outcomes.
Unlike typical security issues caused by coding errors, these vulnerabilities exploit the intended functionality of the application.
Common Examples
- Excessive Trust in Client-Side Controls – Assuming users will interact only through the provided interface, leading to potential bypasses of client-side validations.
- Flawed Assumptions About User Behavior – Designing workflows based on expected user actions without accounting for malicious inputs or sequences.
- Domain-Specific Flaws – Industry-specific logic errors that attackers can exploit due to a deep understanding of the business domain.
Implementing Server-Side Validation in Laravel
Relying solely on client-side validation is a common pitfall. To ensure data integrity, always implement server-side validation in your Laravel applications.
Example
use Illuminate\Http\Request;
use App\Models\Product;
public function store(Request $request)
{
$validatedData = $request->validate([
'name' => 'required|string|max:255',
'price' => 'required|numeric|min:0',
'quantity' => 'required|integer|min:1',
]);
Product::create($validatedData);
}
In this example, the store
method validates the incoming request to ensure all required fields meet the specified criteria before creating a new product.
Avoiding Flawed Assumptions About User Behavior
Designing applications based on assumptions about user behavior can introduce vulnerabilities.
For instance, assuming users will follow a specific sequence of actions may lead to unintended access or data manipulation.
Example
public function updateProfile(Request $request, User $user)
{
if ($request->user()->id !== $user->id) {
abort(403, 'Unauthorized action.');
}
// Proceed with profile update
}
Here, the code ensures that users can only update their own profiles by checking that the authenticated user's ID matches the profile ID being updated.
Preventing Domain-Specific Flaws
Understanding the business domain is crucial to identifying potential logic flaws.
For example, in an e-commerce application, ensuring that discount codes cannot be applied multiple times or combined improperly is essential.
Example
public function applyDiscount(Request $request, Order $order)
{
$discount = Discount::where('code', $request->input('code'))->first();
if (!$discount || $discount->isExpired() || $order->hasDiscountApplied()) {
return response()->json(['error' => 'Invalid discount.'], 400);
}
$order->applyDiscount($discount);
}
This function checks for the existence and validity of a discount code and ensures it hasn't already been applied to the order.
Utilizing Free Website Security Tools
Regularly testing your Laravel application for vulnerabilities is essential.
Use free tools like the Website Vulnerability Analyzer to identify and address potential security issues.
📌 Screenshot of Website Vulnerability Analyzer:
Screenshot of the free tools webpage where you can access security assessment tools.
This tool provides comprehensive security reports to check Website vulnerabilities that highlight detected vulnerabilities and recommendations for mitigation.
📌 Sample Vulnerability Assessment Report:
An Example of a vulnerability assessment report generated with our free tool, providing insights into possible vulnerabilities.
Conclusion
Preventing business logic vulnerabilities in Laravel requires a thorough understanding of application workflows and diligent implementation of server-side controls.
By anticipating potential misuse and regularly testing your application with tools like the Website Vulnerability Scanner, you can enhance your application's security.
For more insights into cybersecurity and pentesting, visit the Pentest Testing Blog.
This content originally appeared on DEV Community and was authored by Pentest Testing Corp
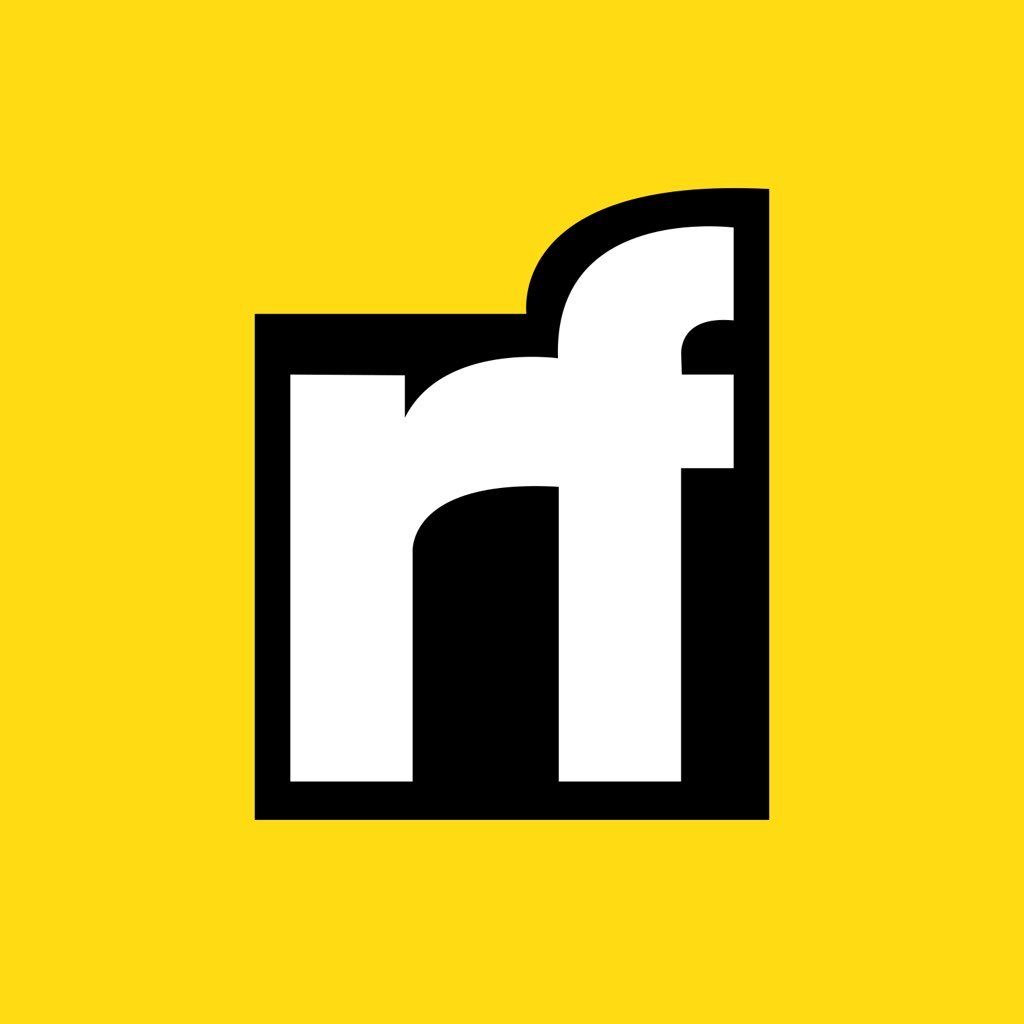
Pentest Testing Corp | Sciencx (2025-02-27T08:56:41+00:00) Prevent Business Logic Vulnerabilities in Laravel. Retrieved from https://www.scien.cx/2025/02/27/prevent-business-logic-vulnerabilities-in-laravel/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.