This content originally appeared on DEV Community and was authored by Putra Prima A
Ever wondered why senior devs choose Collections? The results will surprise you.
Let me take you on a journey through the world of PHP development where code readability battles with performance. As someone who's been in the trenches, I've seen firsthand how the right approach can save your team countless hours of frustration.
The Readability Revolution 📖
The haters have always dragged PHP through the mud for various reasons, but one of the easiest targets has always been how messy the code can look. You might think code "prettiness" is just aesthetic preference, but when you're maintaining large codebases for years, clean code becomes invaluable.
During my time at Apple, I built and maintained a suite of financial applications used across the company. One thing became crystal clear: code that reads like natural language is exponentially easier to onboard new developers into.
Think about it:
Most code forces you to read a substantial chunk, then circle back to the beginning to fully grasp what's happening. It's like reading a mystery novel where you need to flip back to earlier chapters constantly.
// Traditional PHP approach
$filteredData = array_filter($data, function($row) {
return substr($row, 0, 1) === "a";
});
$mappedData = array_map(function($row) {
return $row . "_processed";
}, $filteredData);
$sum = array_reduce($mappedData, function($carry, $row) {
return $carry + strlen($row);
}, 0);
But what if your code could flow like a sentence? 👇
// Laravel Collections approach
$sum = $data->filter(function($row) {
return substr($row, 0, 1) === "a";
})->map(function($row) {
return $row . "_processed";
})->reduce(function($carry, $row) {
return $carry + strlen($row);
}, 0);
See the difference? The Collections approach reads almost like English: "Filter this data, then map it, then reduce it to a sum."
Why Collections Make Your Code Sing 🎵
Laravel Collections transform complicated array operations into an elegant chain of commands. They allow your code to tell a story instead of presenting a puzzle.
When you write:
$result = $users->filter(function($user) {
return $user->age >= 21;
})->sortBy('lastName')->take(5)->pluck('email');
Even a junior developer can understand: "Filter users who are 21 or older, sort them by last name, take the first 5, and get their emails."
This expressiveness isn't just nice-to-have—it's a productivity multiplier. New team members can get up to speed faster, bugs become easier to spot, and maintenance becomes less of a headache.
I'll update that section to clarify that you're referencing someone else's experiments from the Hackernoon article, rather than conducting them yourself.
The Performance Question ⚡
Of course, readability means nothing if your application crawls to a halt. So how do Collections stack up against traditional PHP array functions when it comes to performance?
According to experiments conducted in an article on Hackernoon (https://hackernoon.com/illuminate-collections-vs-php-arrays), where they tested arrays containing 100,000 random strings, executing each function 100 times and calculating the average response times, here's what they discovered:
Operation | PHP Traditional | Laravel Collections | Winner |
---|---|---|---|
Filter | 5.07ms | 6.49ms | PHP ✅ |
Search | 0.79ms | ~0ms | Collections ✅ |
Map | 3.45ms | 4.18ms | PHP ✅ |
Sort | 25.27ms | 11.18ms | Collections ✅ |
Each | 1.03ms | 6.96ms | PHP ✅ |
Reduce | 2.78ms | 7.75ms | PHP ✅ |
Splice | 1.00ms | 0.74ms | Collections ✅ |
The results reveal an interesting pattern. While Collections win decisively in sorting (saving an impressive 14ms per operation) and slightly outperform in search and splice operations, traditional PHP functions take the lead in filter, map, foreach, and reduce operations.
Making Smart Tradeoffs 🧠
The performance differences highlight an important truth about software development: there are always tradeoffs.
When working with large datasets where you'll be doing heavy iteration or reduction, traditional PHP functions might be the way to go. The performance gap for foreach (nearly 7x slower with Collections) and reduce (almost 3x slower) is significant enough to matter in high-throughput applications.
However, if your application involves frequent sorting of large arrays, Collections offer a substantial performance advantage—more than twice as fast as PHP's native sort function.
For operations like filter and map where the performance difference is relatively small (about 20%), the improved readability of Collections might well be worth the minor performance hit.
Real-World Considerations 🌍
Beyond synthetic benchmarks, there are other factors to consider:
Team Familiarity: If your team is already fluent with Collections, switching back to traditional array functions might create more friction than it's worth.
Codebase Consistency: Mixing approaches within the same codebase can be confusing. Sometimes it's better to choose one approach and stick with it.
Application Context: For user-facing operations where a few milliseconds won't be noticed, prioritize readability. For high-volume API endpoints or data processing tasks, optimize for performance.
Future Maintenance: Remember that code is read far more often than it's written. Those extra milliseconds saved today might cost hours of developer time tomorrow.
The Best of Both Worlds 🌈
What I've learned from my experience is that you don't have to choose a side and die on that hill. Smart developers know when to use each tool:
// Use traditional PHP for performance-critical loops
foreach ($largeDataset as $item) {
// Performance-critical operations
}
// Use Collections for complex transformations where readability matters
$report = $transactions
->groupBy('category')
->map(function ($items, $category) {
return [
'category' => $category,
'count' => $items->count(),
'total' => $items->sum('amount'),
'average' => $items->avg('amount')
];
})
->sortByDesc('total');
Practical Guidelines 📝
Based on my testing and real-world experience, here are some practical guidelines:
Default to Collections for most array manipulations—they make your code more expressive and maintainable.
Switch to traditional PHP functions for performance-critical loops and reduce operations, especially with large datasets.
Always use Collections for sorting large arrays—the performance benefit is substantial.
Benchmark your specific use case if you're unsure—synthetic tests are useful but your actual application context matters more.
Consider readability first unless performance is a genuine bottleneck—premature optimization is still the root of much evil.
The Bottom Line 💯
Laravel Collections represent a beautiful evolution in PHP development that aligns with modern programming principles. They transform PHP's somewhat chaotic array manipulation functions into an elegant, chainable API that makes your code more expressive and maintainable.
While there are performance tradeoffs, they're often worth it for the improved developer experience and code clarity. And in cases where performance is critical, you can always fall back to traditional PHP functions for specific operations.
Remember, the goal isn't to write code that runs fast today but to create systems that can be efficiently maintained and enhanced for years to come. Sometimes that means optimizing for human understanding rather than CPU cycles.
Your Turn! 🙋♂️
Are you using Collections in your PHP projects? Have you found them to be a game-changer for readability, or do you stick with traditional array functions for performance reasons?
Drop a comment below sharing your experience—I'd love to hear how other developers are balancing these tradeoffs!
Or if you're struggling with specific code readability challenges in your project, DM me with your questions. I'm always happy to help fellow developers write cleaner, more maintainable code.
Raise your hand if you're ready to give Collections a try in your next project! 🖐️
This content originally appeared on DEV Community and was authored by Putra Prima A
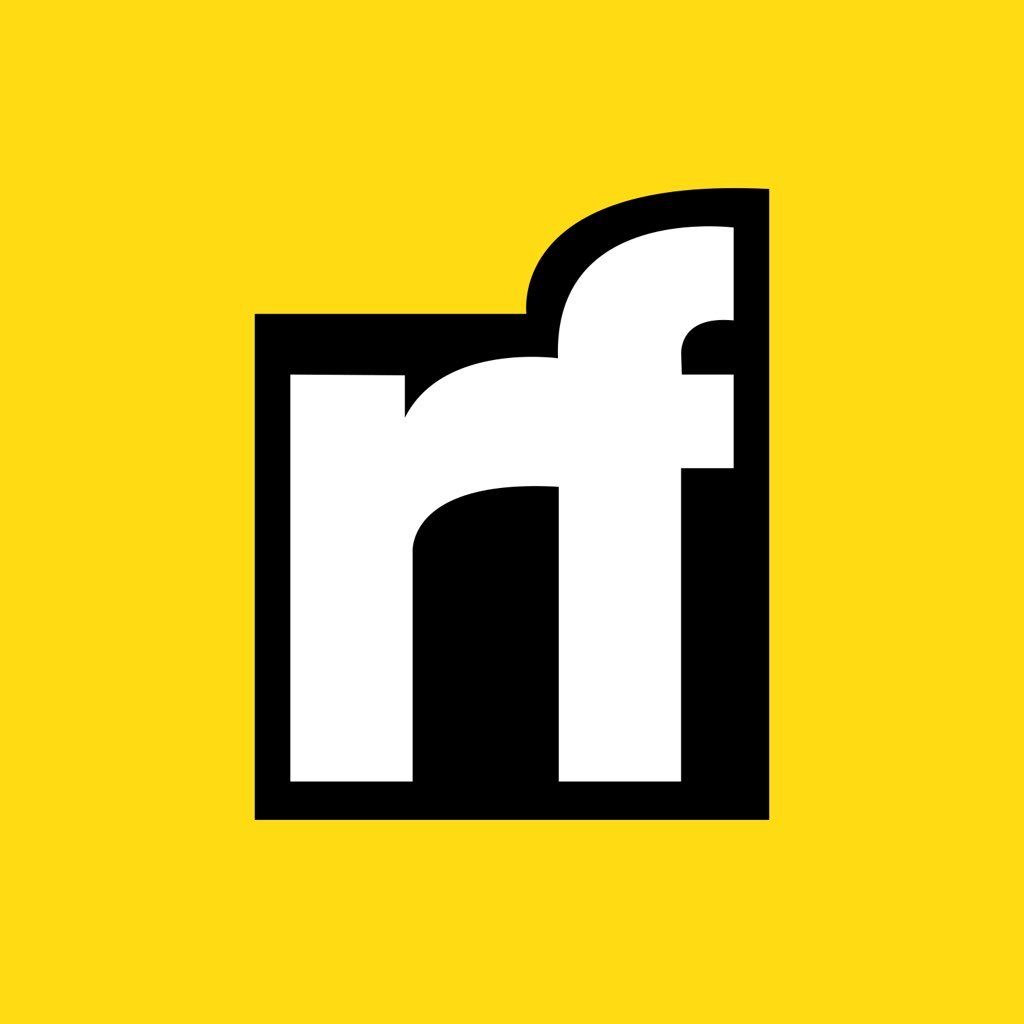
Putra Prima A | Sciencx (2025-02-28T09:00:00+00:00) 7 Reasons PHP Collections Beat Traditional Arrays 🚀. Retrieved from https://www.scien.cx/2025/02/28/7-reasons-php-collections-beat-traditional-arrays-%f0%9f%9a%80/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.